Quick Overview:
In the last three to four years, modern and trendy web apps with real-time communication have become a cornerstone for constructing engaging and interactive user experiences (UX). Enter SignalR, a powerful library in the .NET Core framework that empowers developers to establish real-time connections between clients and servers. Whether it’s instant messaging, live notifications, collaborative tools, or online gaming, SignalR facilitates seamless and bi-directional communication, eliminating the need for the traditional request-response model. This article dives into the world of SignalR, unraveling its key concepts, highlighting its remarkable benefits, and providing illuminating examples that showcase its capabilities.
SignalR on .NET 6 – The Complete Guide
In the dynamic world of software development, the concept of responsiveness takes center stage. End users hold the expectation that applications should swiftly respond in real-time to their interactions. Whether it involves witnessing a new message materialize within a chat interface or observing live updates unfold within a collaborative document, the demand for seamless and immediate reactions remains high. Similarly, receiving instant feedback while immersed in a multiplayer online game also contributes to this persistent demand.
But how can we make this happen? Especially since many of the applications we’ve used follow a conventional pattern, with a client-server architecture forming the fundamental structure. In this setup, the user interface (UI) or client takes the lead by commencing communication. It accomplishes this by dispatching a request to the server, which subsequently handles the request and delivers a corresponding response. While versatile, this setup falls short when rapid server-to-client updates are needed without a specific client request. So, how to overcome this limitation?
Introducing SignalR, a technological breakthrough that shines as a symbol of innovation within this context. It ushers in a new way of thinking by allowing communication to travel from the server to the client, all without the necessity of the client triggering a request. This shift marks a significant change in how communication functions in the software domain, presenting a solution that harmonizes better with human communication styles’ dynamic and diverse tendencies.
With this, I hope you got a fundamental idea of what SignalR is. If not, here’s a crisp explanation.
What is SignalR?
In lay terms, SignalR, found within the Microsoft .NET environment, is a library designed for real-time communication. This library helps (empowers) developers to construct interactive and dynamic web applications by establishing instantaneous connections between the server and its connected clients. The library proves especially valuable in situations where updates from the server to clients must be transmitted without requiring the clients to consistently query the server.
How to Implement SignalR in ASP.NET Core 6 Web API and Angular 14?
The below-mentioned steps outline the implementation process of using SignalR with .NET Core 6 Web API and Angular 14 to create real-time communication between a server and clients.
For better understanding, we have divided it into two parts:
- Backend implementation using .NET Core 6 Web API and SignalR, and
- A client application using Angular 14
Backend implementation using .NET Core 6 Web API and SignalR
1: Create a .NET Core Web API application: Begin by creating the foundation of your backend application.
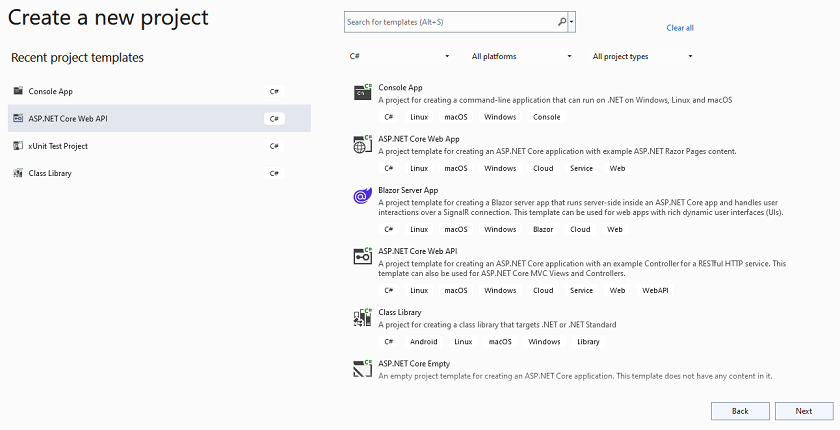
2: Configure your project: Set up the project configuration for further development.
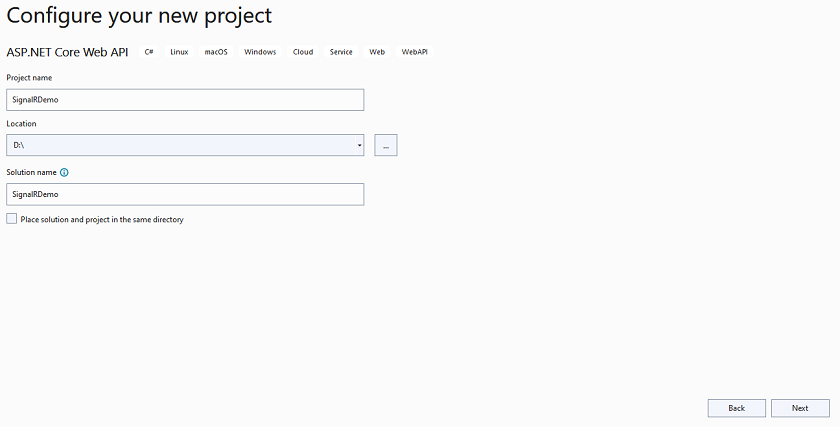
3: Additional Information: Add some additional information about your project.
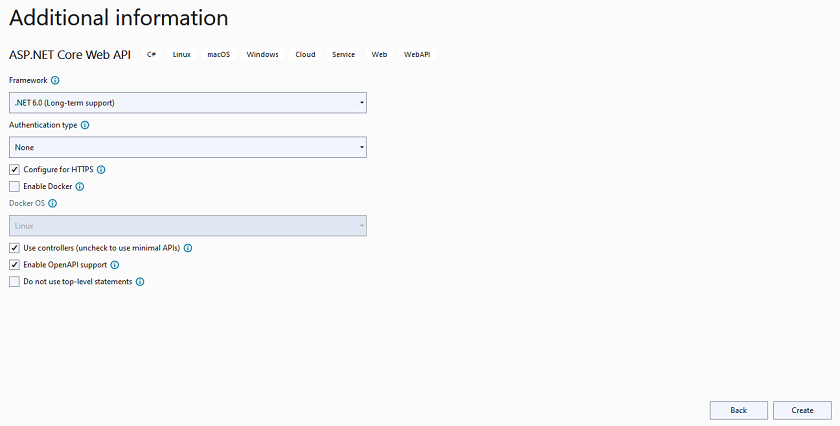
4: Install SignalR NuGet package: Integrate the SignalR library into your project to enable real-time communication.
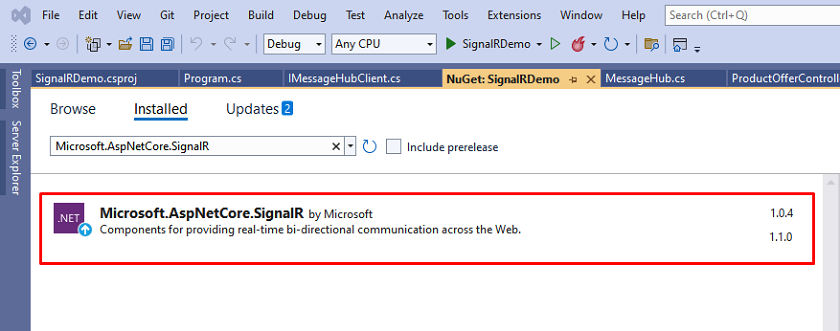
5: Project Structure: Provide an overview of how the project is structured.
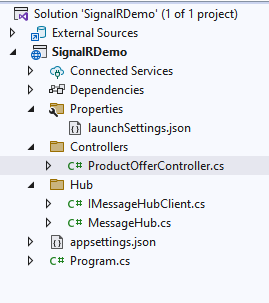
6: Define IMessageHubClient interface: Create an interface inside the “Hub” folder, outlining the methods for sending offers to users.
namespace SignalRDemo.Hub { public interface IMessageHubClient { Task SendOffersToUser(List < string > message); } }
7: Implement MessageHub class: Develop the actual SignalR hub that extends the IMessageHubClient interface. This class manages to send offers to clients.
using Microsoft.AspNetCore.SignalR; namespace SignalRDemo.Hub { public class MessageHub: Hub < IMessageHubClient > { public async Task SendOffersToUser(List < string > message) { await Clients.All.SendOffersToUser(message); } } }
8: Create ProductOfferController: Design a controller responsible for handling product offers. Inject the IHubContext to enable sending messages via SignalR.
using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.SignalR; using SignalRDemo.Hub; namespace SignalRDemo.Controllers { [Route("api/[controller]")] [ApiController] public class ProductOfferController: ControllerBase { private IHubContext < MessageHub, IMessageHubClient > messageHub; public ProductOfferController(IHubContext < MessageHub, IMessageHubClient > _messageHub) { messageHub = _messageHub; } [HttpPost] [Route("productoffers")] public string Get() { List < string > offers = new List < string > (); offers.Add("20% Off on IPhone 12"); offers.Add("15% Off on HP Pavillion"); offers.Add("25% Off on Samsung Smart TV"); messageHub.Clients.All.SendOffersToUser(offers); return "Offers sent successfully to all users!"; } } }
9: Register services and CORS policy: In the Program.cs class set up services related to SignalR and Cross-Origin Resource Sharing (CORS) policy.
using SignalRDemo.Hub; var builder = WebApplication.CreateBuilder(args); // Add services to the container. builder.Services.AddSignalR(); builder.Services.AddCors(options => { options.AddPolicy("CORSPolicy", builder => builder.AllowAnyMethod().AllowAnyHeader().AllowCredentials().SetIsOriginAllowed((hosts) => true)); }); builder.Services.AddControllers(); // Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle builder.Services.AddEndpointsApiExplorer(); builder.Services.AddSwaggerGen(); var app = builder.Build(); // Configure the HTTP request pipeline. if (app.Environment.IsDevelopment()) { app.UseSwagger(); app.UseSwaggerUI(); } app.UseCors("CORSPolicy"); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); endpoints.MapHub < MessageHub > ("/offers"); }); app.UseHttpsRedirection(); app.MapControllers(); app.Run();
10: Run the application: Execute the application, and you should see the Swagger UI and API endpoints for sending messages.
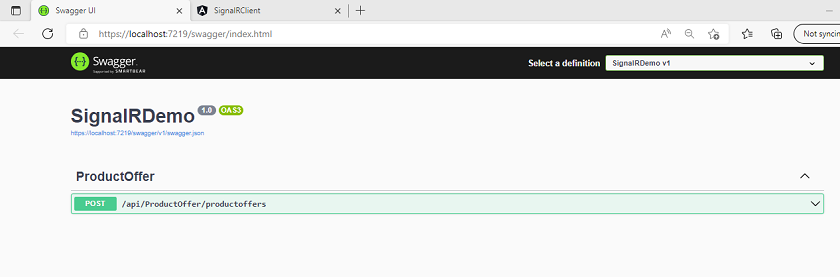
A Client Application using Angular 14?
1. Create an Angular application: Begin by generating an Angular application named “SignalRClient.”
ng new SignalRClient
2. Install SignalR library: Add the SignalR library to your client application to enable interaction with the server.
npm install @microsoft/signalr --save
3. Install Bootstrap: Integrate Bootstrap to enhance the user interface of the application.
ng add @ng-bootstrap/ng-bootstrap
Then, include the bootstrap script within the scripts and styles section of the angular.json file.
"styles": [
"src/styles.css",
"./node_modules/bootstrap/dist/css/bootstrap.min.css"
],
"scripts": [
"./node_modules/bootstrap/dist/js/bootstrap.min.js"
]
4. Configure app.component.ts: In this TypeScript file, create a HubConnection instance, set up the connection with the server, and define the logic to receive and display offers.
import { Component } from '@angular/core'; import { HubConnection, HubConnectionBuilder, LogLevel } from '@microsoft/signalr'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'SignalRClient'; private hubConnectionBuilder!: HubConnection; offers: any[] = []; constructor() {} ngOnInit(): void { this.hubConnectionBuilder = new HubConnectionBuilder().withUrl('https://localhost:7219/offers').configureLogging(LogLevel.Information).build(); this.hubConnectionBuilder.start().then(() => console.log('Connection started.......!')).catch(err => console.log('Error while connect with server')); this.hubConnectionBuilder.on('SendOffersToUser', (result: any) => { this.offers.push(result); }); } }
5. Create app.component.html: Add the HTML snippet to portray (display) the offers acquired from the server.
<h2>Data loaded from the Web API:</h2> <div *ngIf="offers.length>0" class="alert alert-warning" role="alert"> <li *ngFor="let item of offers"> Offers: {{item}} </li> </div>
6. Run the client app: Now, utilize the npm start command to initiate the client application.
npm start
7. Access the client app: In a browser, open the client application URL (http://localhost:4200/) to view the application interface.
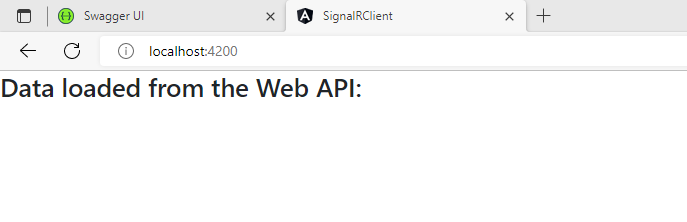
8. Hit the server API endpoint: Use the Swagger UI of the backend application to send offers to clients.
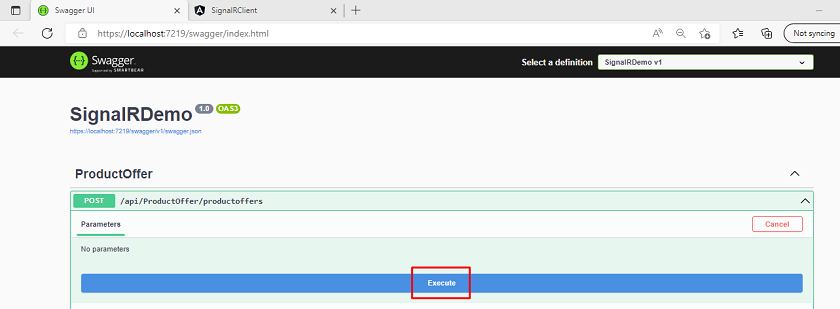
9. See the offer message: After hitting the server API endpoint, the offer message should be displayed in the client application.
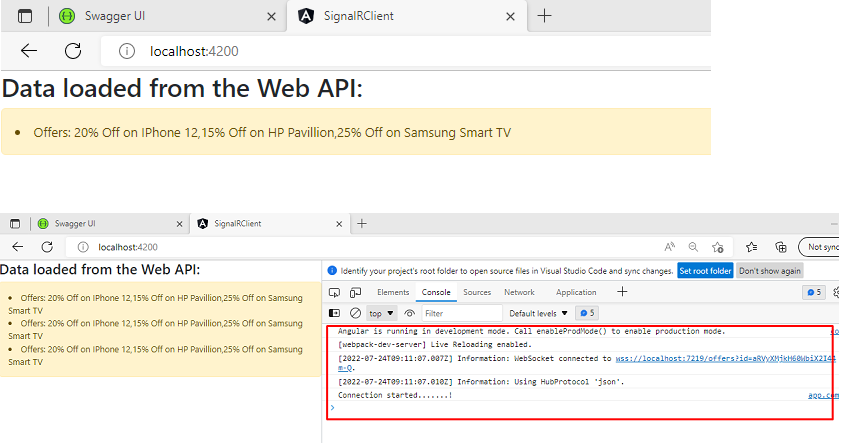
The steps above provide a comprehensive guide for implementing real-time communication using SignalR in ASP.NET Core Web API and Angular client application. It covers both the backend and frontend aspects of the implementation, detailing the setup, configuration, code creation, and final execution.
Examples of SignalR ASP.NET Core Usage
- Real-Time Chat Application: Construct a chat platform where users can instantaneously engage in live conversations, sending and receiving messages without manually refreshing the page.
- Live Notifications: Establish a notification system that promptly informs users about significant events, like new emails, friend requests, or system upgrades.
- Collaborative Drawing App: Develop an application for collaborative drawing, enabling “n” number of users to sketch on a shared canvas simultaneously in real time.
- Stock Market Updates: Generate a dashboard that provides traders and investors with minute-by-minute updates on stock prices and market trends, ensuring they stay well-informed and up-to-date.
- Online Gaming: Design multiplayer games that demand real-time interaction and synchronization among players for an immersive gaming experience.
Key Concepts of ASP.NET Core SignalR
- Hubs: A hub is a high-level programming model in SignalR that abstracts the low-level communication details. It provides methods that clients can call and broadcast messages to all connected clients.
- Groups: It allows clients to be grouped together, enabling targeted message delivery to specific sets of clients.
- Persistent Connections: It supports both persistent connections and WebSockets, providing flexibility in real-time communication between the server and clients.
- Connection Management: It manages the lifecycle of connections, handling connection establishment, reconnection, and disconnection scenarios gracefully. This helps maintain a stable communication channel even in the face of network disruptions.
- Transport Protocols: SignalR automatically chooses the best transport protocol available, which can include WebSockets, Server-Sent Events, and long polling, depending on the capabilities of the client and server.
Advantages of Using SignalR .NET Core
- Bi-Directional Communication: SignalR facilitates your application with bi-directional communication capabilities, i.e., not only can the server send data to clients, but clients can also send data back to the server in real-time. This opens up possibilities for dynamic interactions and seamless exchanges of information.
- Creating Real-Time Chatroom Applications: SignalR shines when it comes to building real-time chatroom applications. With its ability to instantly push messages to participants, you can create chat platforms where messages appear in real-time as they’re sent, fostering lively conversations and engagement.
- Cost-Free Solution: One significant perk of SignalR is that it’s free. You can combine its powerful real-time communication features into your .NET Core application without extra cost, making it a budget-friendly choice.
- Fallback to SSE (Server-Sent Events): It is built to adapt. If newer transport mechanisms like WebSockets aren’t available, SignalR gracefully falls back to older ones, such as Server-Sent Events. This ensures compatibility across different browsers and platforms, guaranteeing a smooth experience for all users.
- User-Friendly Implementation: It is designed with simplicity in mind. Its user-friendly API and intuitive concepts make it moderately straightforward to incorporate real-time functionality into your app. This reduces the learning curve for developers and accelerates the development process.
- Broadcasting Messages: SignalR makes broadcasting messages a breeze. You can send messages to specific or multiple clients simultaneously as needed. This opens doors to scenarios like targeted notifications, group chats, and global announcements.
Let’s Design the Perfect ASP.NET Application for Your Enterprise Business
Bring your unique software & web application vision to a team of ASP.NET experts for Enterprise business. Our dedicated .NET developers design and build custom .NET app solutions for your needs.
Conclusion on SignalR in ASP.NET Core
At its core, SignalR simplifies implementing real-time web functionality by handling compatibility across browsers and platforms. It supports modern transport mechanisms like WebSockets and Server-Sent Events and fallback options like long polling when needed. This allows your .NET Core developer to choose the most appropriate transport based on client capabilities and network conditions. The versatility of SignalR empowers your developer to ensure a consistent real-time experience for all your users. So, when looking to hire .NET Core developers with SignalR experience for implementing robust and seamless real-time communication in your web apps.
Expert in Software & Web App Engineering
Parag Mehta, the CEO and Founder of Positiwise Software Pvt Ltd has extensive knowledge of the development niche. He is implementing custom strategies to craft highly-appealing and robust applications for its clients and supporting employees to grow and ace the tasks. He is a consistent learner and always provides the best-in-quality solutions, accelerating productivity.