Quick Overview:
Several developers came across the React side-effect while doing ReactJS application development or learning about it. And some even confuse it with a kind of bug or an error.
However, it’s a feature that aids in extending the functionality of the application. In addition, learning about side effects and handling them would help improve app robustness. And as per professionals, it’s as essential as other React components.
Here, you will understand the basics of React side effects and how to efficiently handle them in a ReactJS app. So, let’s get started.
What is React Side-Effect?
Before you dive into the React Side-Effect, you must know about the pure function. It would help you gain a deep insight into the working and handling of the side-effect.
In ReactJS, almost every component is a pure function. It means that their output would be the same for the same input. And you can determine the output from the input value. In addition, all such pure components work inside the React environment and don’t communicate or fetch data from an external source. But, ReactJS provides the capability to develop an application that can:
Insight To React Side-Effect In Render
In every ReactJS-based application, a side-effect gets used in one way or another. It helps to manage the unexpected results from the system and provide an efficient output to the user. Also, the code puts side-effect components separately, especially away from the rendering process.
Whenever you write a ReactJS code, always remember to configure side-effect execution after the rendering process. Otherwise, it can interrupt the rendering, leading to quality and performance downgrade.
Further, there’s a common mistake while defining the side-effect that most developers do. They don’t use a dependency array with it, which leads to run side-effect after every render. In the other section, we will discuss handling side-effect. Then, you will gain a better insight into the relation and implementation of side effects and rendering.
However, it entirely depends upon the requirements of whether someone wants side-effect to execute once or after every render. But, it’s always recommended to execute it only one time and too after the rendering procedure.
Using the useEffect To Handle React Side-Effect
In ReactJS application development, using the useEffect hook is the most prominent way to handle side effects. It’s a hook that lets developers process side effects for updating DOM, fetching data, and using timers. And every useEffect needs two arguments to operate.
Syntax of useEffect:
useEffect(<function>, <dependency>)
The <function> argument in the syntax is mandatory; however, you can skip to provide a variable for the <dependency> argument.
Furthermore, you must understand the following concepts about the control flow of the useEffect:
- The ReactJS components are based on state, change of context, and prop for re-rendering.
- If multiple useEffect hooks are defined in the code, then ReactJS will analyze and cross-verify all with the current condition. And the best one aligning with the conditions will get processed.
- The second argument, i.e., <dependency>, is an array from the state, context, or props components.
- The scheduling and execution of every side-effect depend upon the definition of its dependencies.
- If you don’t provide a dependency argument in the useEffect syntax, that particular effect will be processed after each rendering.
Additionally, it would be best to use the useEffect hook’s cleanup functionality. It aids in minimizing memory leaks for side effects that are not needed further. You must utilize it for timeouts, event listeners, and similar services.
However, before you start implementing the useEffect hook, focus on the associated rules:
- You can only invoke the useEffect hook from the top-level function. And that function must contain your React component.
- You cannot call useEffect hooks within the nested code and inside another function.
- You can create custom useEffect hooks as per your requirement. But, the condition is the same, as you can only call a hook from the top-level function.
Once you understand all three rules, utilizing useEffect and handling side-effects will become effortless. And your ReactJS application will seamlessly connect with external sources through APIs for data-oriented operations.
Now, let’s look at the code to define the useEffect hook.
useEffect( () => { // execute side effect }, // optional dependency array [ // 0 or more entries ] )
And, if you don’t want to pass the <dependency> argument, use the following signature.
useEffect(() => { // execute side effect })
To understand the useEffect usage more thoroughly, let’s create an example code of changing the title through an input box. The code’s primary function is to modify the page title to the same as the input entered by an end-user.
The ReactJS Code:
import React, { useState, useRef, useEffect } from "react"; function EffectsDemoNoDependency() { const [title, setTitle] = useState("default title"); const titleRef = useRef(); useEffect(() => { console.log("useEffect"); document.title = title; }); const handleClick = () => setTitle(titleRef.current.value); console.log("render"); return ( <div> <input ref={titleRef} /> <button onClick={handleClick}>change title</button> </div> ); }
In the provided above code, you can see that useEffect gets defined with only the first mandatory argument. It means that it will execute after every rendering. You must execute this code on your machine to dig deeper into the concept.
Moreover, the code contains a console.log statement, enabling one to view the side-effect working by analyzing the logs. And in the logs, you will see that first, the ReactJS code is rendering, and then the useEffect hook is coming into action.
Let’s see more examples based on different situations, execution after the first rendering and execution after every rendering.
Code example for useEffect processing after first rendering.
With this code, you can enable the ReactJS application to update the loadData directly after the first rendering.
const { useState, useEffect } = React; function App() { const [loading, setLoading] = useState(false); const [data, setData] = useState(null); function loadData() { setLoading(true); setTimeout(() => { setLoading(false); setData([1, 2, 3, 4, 5]); }, 1000); } useEffect(loadData, []); return ( <> {loading && <p>Loading...</p>} {data && <pre>{JSON.stringify(data, null, 1)}</pre>} </> ); } ReactDOM.createRoot(document.getElementById('root')).render(<App />);
In most cases where you don’t pass the dependency argument, the useEffect hook gets executed after every rendering. In the code example, in which the user inputs the title, it’s possible to face such a problem. Thus, to eliminate it, you must pass an empty dependency or array. As a result, the ReactJS application will function as per requirements.
Also, you must ensure that you don’t use the side-effect functionality if the application needs to transform data for rendering purposes. And for handling and managing user events. For rest operations, the side-effect is a reliable React component.
An Advanced Helping Hand in Handling React Side-Effect
For the fast pace ReactJS Application Development, you can utilize the ESLint plugin, a Hooks API component. It integrates with IDE and helps maintain the app with useEffect hook rules. Also, it aids in eliminating bugs and streamlining the passing of dependency arguments.
Moreover, you can also rely on it for providing the most reliable array, ensuring the smooth execution of side-effect after the rendering. Once you start utilizing the ESLint plugin, it will automatically enforce the hook rules, reducing your efforts and development time.
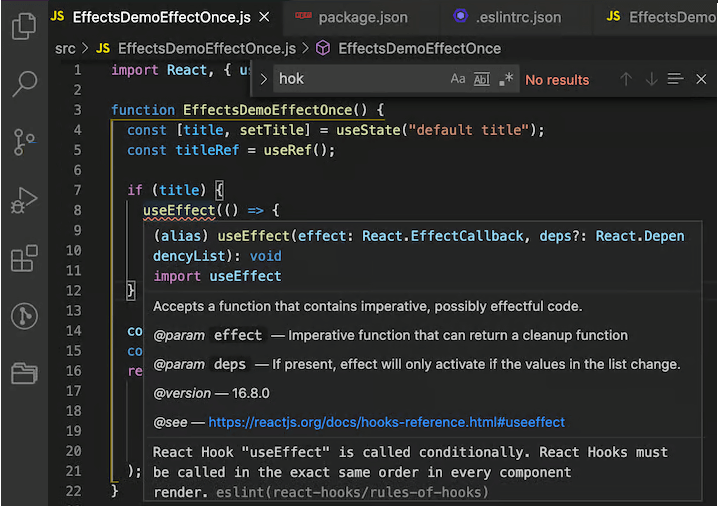
Additionally, take care of the following points while using the plugin:
- View and analyze the dependency suggestion provided by the plugin before implementing it.
- Thoroughly test the code to resolve compatibility issues.
- Firstly, pass dependencies manually and take the plugin’s suggestion as a secondary source.
ReactJS Development Services, For Splendid Business Applications
ReactJS applications are prevalent in every industry. And most companies prefer it over other technologies. But, you can only leverage its benefits if you select an authentic ReactJS development company, such as Positiwise Software Pvt Ltd.
Empower Your Web Presence with Expert ReactJS Development Services!
Unlock unparalleled web experiences with our top-tier ReactJS Development Services. Our team of seasoned experts is dedicated to crafting dynamic, high-performance web applications that captivate users and drive business growth.
By partnering with Positiwise, you can ensure a top-notch ReactJS app complying with industry standards. In addition, You can take advantage of:
- Responsive UI Creation Service
- Custom ReactJS Development Service
- Native Web App Development
- ReactJS Maintenance and Support
- Plugin Development
- App Migration Services and more
Thus, you can avail of all required services only in one place by selecting Positiwise as your technology partner. In addition, it also guarantees topmost data security, timely delivery, and affordable development.
Concluding Up
React side-effect is a crucial component of a ReactJS application. It helps establish communication with external resources, such as local storage and supports utilizing APIs. However, one should crucially handle them using the useEffect hook; otherwise, it can lead to an application crash in the middle. The useEffect syntax consists of two primary arguments, the dependency, and the function. Among both, dependency is optional, but declaring a function is a must.
Furthermore, you must always declare both arguments. Otherwise, it can cause bugs and errors. And if you want some automated tool to suggest the suitable dependency array, then utilize the ESLint plugin. Moreover, learn the useEffect hook rules before you implement them.
Expert in Marketing Strategy and Brand Recognition
Jemin Desai is Chief Marketing Officer at Positiwise Software Pvt Ltd, he is responsible for creating and accelerating the company’s marketing strategy and brand recognition across the globe. He has more than 20 years of experience in senior marketing roles at the Inc. 5000 Fastest-Growing Private Companies.