Quick Overview:
Consuming API is a crucial function of any .NET application. While providing .NET development services, developers must focus on selecting the suitable mechanism. Otherwise, it can impact the performance and efficiency of the software. In the .NET ecosystem, HttpClient and RestSharp are the two most popular mechanisms used for consuming APIs. However, some say both are equally compatible, but some .NET developers prefer one.
But, here, you will get your answer on whether HttpClient is better or RestSharp. So, let’s get started.
HttpClient or RestSharp: best alternative for .NET framework development
What is HttpClient?
HttpClient is a built-in class of the .NET development framework that enables sending and receiving HTTP requests and responses. All the requests and responses get transmitted from the resources, defined as a URL.
For instance, in C# (C Sharp), it gets defined as:
Public class HttpClient: System.Net.Http.HttpMessageInvoker
Further, the instance of the HttpClient class functions as a session for sending HTTP requests from its connection pool. Every such instance has its pool of connections, and each has its processing environment. Therefore, an isolated ecosystem gets created for instances to execute the HTTP requests in their pool.
In addition, connection pooling used by HttpClient helps to improve the application performance. When a connection gets established using the HttpClient instance, it doesn’t get terminated after a single request execution. It enables the user to use the same link to send multiple requests and receive responses. Due to this, the load on sockets and resource utilization decreases, accelerating the speed and stability.
Moreover, you don’t have to worry about the platform while using HttpClient, as it is cross-platform compatible. Whether it’s Windows, Mono, Android, iOS, Xamarin, Linux, .NET Core 2.1, or any other, it runs smoothly with all.
The Usage of HttpClient in .NET Development Services
While providing .NET development services, you can utilize HttpClient for multiple purposes, such as:
- To create a connection pool, increasing the application speed and saving additional resource utilization.
- To communicate with Web APIs and streamline the communication to and from a console .NET application.
- To send GET, POST, and PUT requests to the defined URL and get responses in the form of asynchronous operations.
- To receive Web API responses from different .NET applications, such as Windows Service and Form-based and web-based applications.
- To resolve the DNS entries at initiating the connection request made by a client device.
Build Your Business with Custom .NET Application Development Services
Take your business online with custom ASP.NET app development services. Our top .NET developers deliver secure, scalable web applications to grow your enterprise business.
Pros of Using HttpClient
The benefits of using the HttpClient class include the following:
- It’s an in-built .NET framework class, reducing the efforts of implementing it in the application.
- It’s a traditional mechanism of calling Web APIs, and you get Microsoft support.
- HttpClient improves the overall app performance.
- You will get plenty of resources to troubleshoot and resolve its errors from the .NET community.
Cons of Using HttpClient
The disadvantages of the HttpClient class include the following:
- To implement HttpClient, you need to know the core and advanced concepts of the .NET framework.
- It doesn’t support complete serialization and deserialization.
- Primarily, it enables the use of only asynchronous Web API calls.
- Instantiating it for each request can lead to fast exemption of resources.
Recommended Read: Vue vs Angular: Which Framework to Choose?
What is RestSharp?
RestSharp is a third-party library developed for the .NET framework to implement HTTP request and response functioning. It’s a REST API library that gets wrapped around HttpClient and provides the following functions:
- It serializes the payload sent to JSON and XML.
- Aids configuring the content headers, including disposition, type of content, and similar. It supports handling the responses coming from the remote endpoint.
- It also enables the performance of JSON and XML response deserialization.
- It helps add a query, form, header, and similar parameters to the request without extra effort and complications.
If you want to start with RestSharp, the first step is creating an instance. It also uses instances just like HttpClient to make requests. However, by default, it gets set to the GET, which you can modify using the Method parameter.
In addition, RestSharp automatically encodes the GET, POST, or PUT requests. It also adds parameters to them, which will be added with each HTTP request. Besides, you can use it for adding a URL segment and cookies method to retrieve cookie data from the response. Thus, RestSharp aids in customizing every HTTP call aspect and supports sending objects as a request.
The Usage of RestSharp by a .NET Development Company
The primary usage of the RestSharp client library in the .NET framework is as follows:
- It gets used with Blazor-based applications to request external endpoint APIs.
- You can use it to configure the serialization of XML and JSON using the built-in methods.
- Using Request Token, Access Token, 0-legged OAuth, OAuth2, JWT, and custom authenticator mechanism to use authenticators for passing account credentials.
- RestSharp also gets used for handling network errors in case the server is down or the DNS lookup is failing. In addition, you can configure it to throw exceptions while making the request or receiving a response with failed HTTP status.
Pros of Using RestSharp
You can avail of the following benefits by using RestSharp in your .NET application:
- Quick and easy configuration in the .NET application.
- It optimizes app performance and support to provide a seamless experience to users.
- Enables to throw exceptions for network-related issues and errors.
- Allows to customize every parameter of the HTTP call.
- It provides both serialization and deserialization of XML and JSON.
Cons of Using RestSharp
The cons of RestSharp include the following:
- It’s a third-party library, which can cause the .NET application to have additional vulnerabilities.
- No support from Microsoft for resolving its errors.
- It provides the same function as HttpClient.
- You can face complexities with it if Microsoft modifies its architecture in the future.
RestSharp Alternatives: More Comfort To Your .NET Development
As RestSharp is a third-party API client library, multiple alternatives are available. The security risk with the other libraries would be the same. But you can select and implement them according to your comfort and requirements. The RestSharp alternative includes the following:
- Ocelot
- Refit
- Flurl.Http
- FastEndpoints
- EasyHttp
- RestEase
- WebApiClient
- Http.fs
- Tiny.RestClient
- FluentUriBuilder
- Cashew
- HttpClientGoodies
- Apizr
Besides the listed alternatives, if you find any other library or mechanism for a similar purpose, assess its compatibility before implementation. It will aid you in retaining the .NET application performance and quality.
The Performance Difference
Before selecting any mechanism for calling APIs, analyzing their performance is essential. To test HttpClient and RestSharp efficiency, a C# program is used, which provides a static value in response and increases the value by one. With each request, the value increases. The primary aim of this testing is to analyze the time taken by HttpClient and RestSharp to send requests and receive a response.
The C# Code
public static int x = -1;
[Route("test")]
[HttpGet]
public IHttpActionResult Get()
{
x = x + 1;
return Ok(x);
}
Test Case 1: Sending 10 Requests
The output after sending ten requests provides the time taken by HttpClient to be 15 milliseconds. And RestSharp took 59 milliseconds.
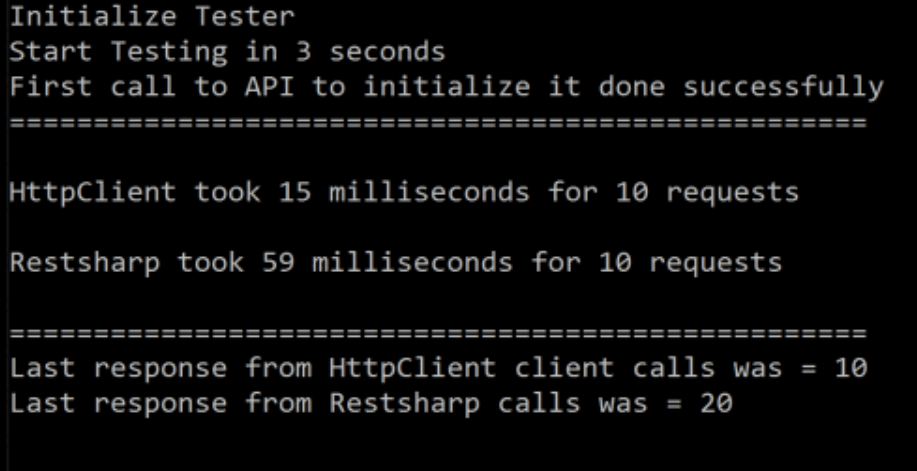
Test Case 2: Sending 500 Requests
In the second test case, HttpClient takes only 494 milliseconds. Whereas, RestSharp took 1079 milliseconds to execute 500 HTTP requests
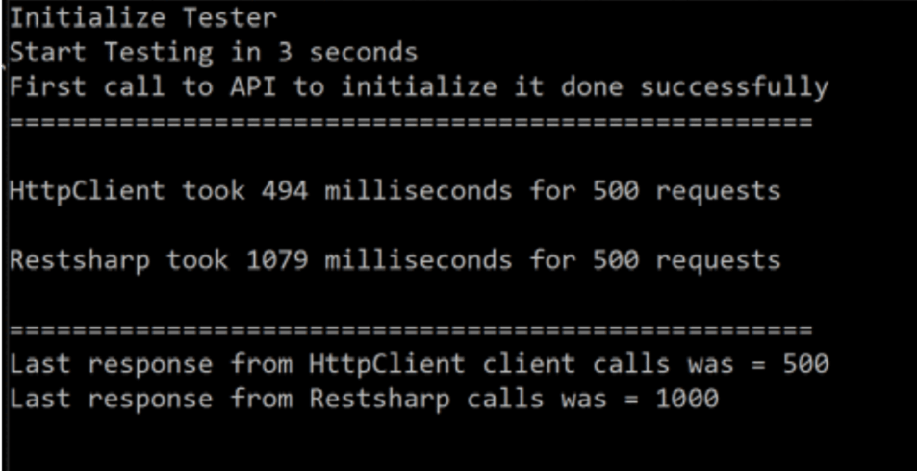
Test Case 3: Sending 5000 Requests
In the third scenario, the difference between time performance increases massively. HttpClient took 4606 milliseconds to process 5000 requests, and RestSharp took 8488 milliseconds.
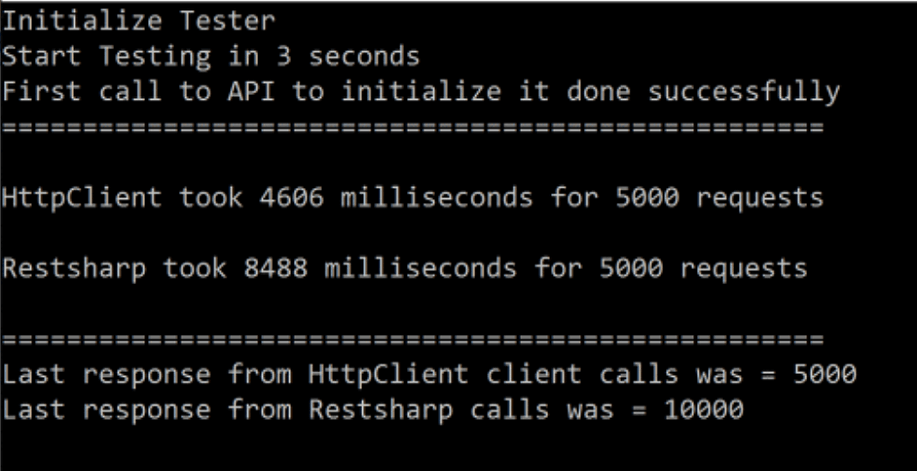
The Final Conclusion: Which One To Choose?
Whether it’s HttpClient or RestSharp, both get used to calling API and transferring HTTP requests and responses. HttpClient is a built-in class, whereas RestSharp is a third-party library. From a security perspective, HttpClient must be used, as it comes directly from Microsoft, and you will receive support for it. On the other hand, RestSharp can create vulnerabilities if the provider doesn’t update it.
Furthermore, as seen in the results of test cases, RestSharp is taking more time to send requests and receive responses. Therefore, you must prefer HttpClient, as its processing time is less, and it gets backed by Microsoft.
Expert in Software & Web App Engineering
Parag Mehta, the CEO and Founder of Positiwise Software Pvt Ltd has extensive knowledge of the development niche. He is implementing custom strategies to craft highly-appealing and robust applications for its clients and supporting employees to grow and ace the tasks. He is a consistent learner and always provides the best-in-quality solutions, accelerating productivity.