Quick Overview:
A common type system is the main component that supports the developers to use multiple programming languages in a single dotnet project. This blog helps you understand the fundamentals of CTS by providing insight into its features and core mechanisms. In addition, you will learn the practical implementation of viewing the CTS output, leading to learning how the code of C# and VB are treated equally and integrated easily.
Common Type System (CTS)
When we talk about .NET interoperability, the CLR is the primary component that comes to our mind. But, under it, the common type system is the one responsible for managing different languages and ensuring their compliance with required protocols. It helps .NET developers or anyone associated with the dotnet application to use multiple languages for it.
Here, we are going to explore the CTS to understand its main mechanisms and its output after processing the code. So, let’s get started.
What Does Common Type System Means?
Common Type System is a crucial component of the common language runtime, which is a .NET framework component. It’s responsible for handling the code compilation and executing it on a compatible device or operating system.
Under the CLR, you find the common type system, which defines the rules, regulations, and protocols for the programming languages. Further, when these rules are followed, developers are able to leverage interoperability. As a result, your application logic written in C# is able to call functions in F# and visual basic and vice versa.
The key concepts that support the functioning of a common type system are as follows:
1: Type Safety: The type safety feature of CTS ensures that multiple languages are aligned with the standards to provide interoperable functionality. Due to this, you will have reduced errors, leading to an improved reliability of CLR and your application.
2: Metadata: This component’s main function is during the runtime, as it offers the details about the type. In addition, reflection is also provided with metadata, which helps dotnet developers inspect and dynamically enable assembly loading and late binding.
3: Type Definition: When the CTS comes into action for enabling interoperability, it provides all the definitions in the form of metadata. Further, this metadata gets utilized by the programming languages to avail of details, such as properties, methods, fields, type names, and more.
4: Value and Reference Types: In CTS, all the types are categorized under value or reference. The value types directly store the data, whereas the reference type points to the data through a reference variable.
In addition, the most common examples of value types are int, structs, and floats. On the other hand, reference type examples are delegate, class, and interface.
The CTS Offerings: Core Features and Functionalities
The features of the common type system are the core supports in enabling interoperability in .NET. These features also execute some of the core operations as listed below.
1: Type Compatibility: When you enable the CTS in your .NET project, it provides you with suggestions to align programming languages with defined protocols. Further, when you align them with the suggestions, the components of different languages are able to integrate and provide you with the required app functionality.
2: Common Intermediate Language: When the source code is provided to the CLR, firstly it gets converted to the intermediate language. It means that all the code written in different languages is now at the same level. Following it, the CTS ensures that compiled code follows the type safety and other instructions.
3: Unified Type System: For enabling interoperability, CTS forces each language to conform with the defined type system. It helps the developers to inherit from a F# class in a C# or VB program. As a result, the classes are impeccably passed, and you are able to integrate the entire codebase into one.
4: Exchange of Metadata: The metadata generated by CTS is compiled with the source code. All the assemblies that run in the program use this metadata to understand the methods, properties, classes, and fields of multiple languages. And it results in running the .NET application built using C#, F#, and VB together or with any of the combo.
5: Interface Implementation: The interface implementation of a common type system leverages you to implement more than one interface for contract-defining purposes. In addition, it also allows to use of interface-based programming, which helps in improving the interoperability and user experience.
The Practical Illustration of CTS Working
In this section, we are going to explore the working of a common type system in .NET environment. We will be creating a project in Visual Studio to illustrate that C# and VB code follow the same type of system when converted using the CLR. You only need to follow the below steps to avail of the insight.
Step 1: Open Visual Studio IDE and create a project with C# class library. Further, generate a class file under the project and name it “Calculator.cs”.
Step 2: Add the code to the “Calculator.cs” file created in the C# project.
namespace CsharpClassLibrary
{
public class Calculator
{
public int Calculate()
{
int a = 10, b = 20;
int c = a + b;
return c;
}
}
}
Step 3: Under the same project add a new VB class library. Now, create a new class file under it with the name “Calculator.cs”.
Step 4: Add the below code to “Calculator.cs” under the VB class library.
Public Class Calculator
Public Function Calculate() As Integer
Dim a As Integer = 10
Dim b As Integer = 10
Dim c As Integer
c = a + b
Return c
End Function
End Class
Step 5: Open the Visual Studio command line with administrative access and run the commands as displayed below. It will run the two instances, handling the C# and VB code.
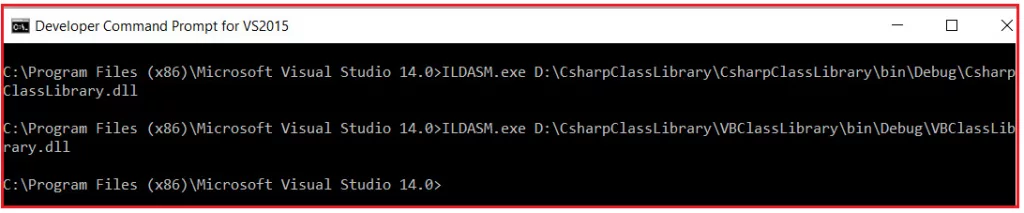
Step 6: Open the IL code of both class library projects. You will see that both are converted to the same type, which is “Int32”. Regardless of the defined variable type in source code, CTS helped them comply with required standard.
Further, as C# and VB code are converted to the same type, it’s obvious that you can use them together and interoperate their logic.
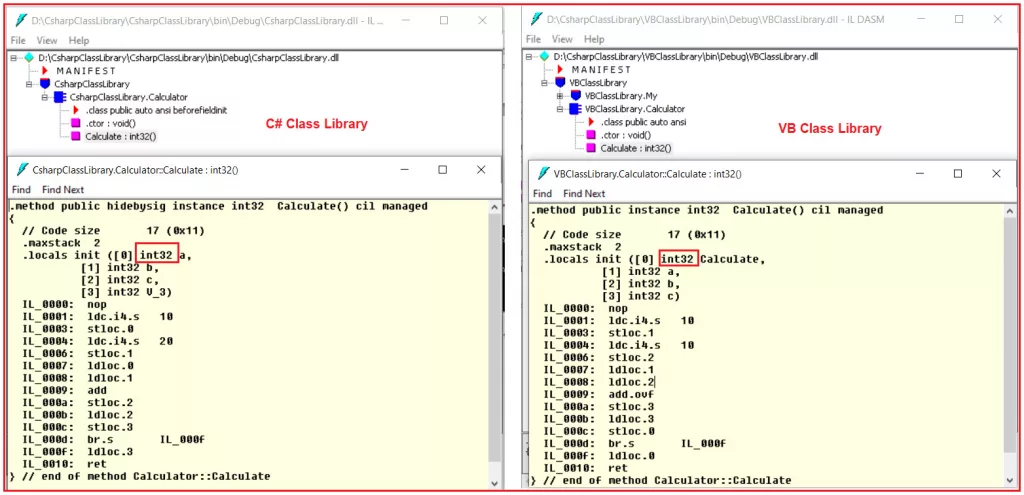
Concluding Up
The common type system is a primary pillar for enabling interoperability in .NET. It helps you define the rules and regulations for programming languages to interact with each other. In addition, some of its main operations supporting interoperability. It include the type of safety, metadata exchange, interface implementation, type definition, and more. Last but not least, you can view its working and final result through the provided practical implementation for better insight. It’ll help you understand, what’s actually happening in the background.
Expert in Software & Web App Engineering
Parag Mehta, the CEO and Founder of Positiwise Software Pvt Ltd has extensive knowledge of the development niche. He is implementing custom strategies to craft highly-appealing and robust applications for its clients and supporting employees to grow and ace the tasks. He is a consistent learner and always provides the best-in-quality solutions, accelerating productivity.